Demystifying Class in JavaScript
Classes in JavaScript are a hurdle for many. Many complaints about the different behavior of a class in JS. In this post, I’ll try to explain how a class works in JS and how to achieve class like behavior without using class keyword.

People from object-oriented background often get confused with classes in JS. Often people come with some assumptions and try to relate things with each other which is a good thing to fast track our understanding. But while trying to relate the behavior of a class in Java or C++ to class in JS puts us in more confusion rather than simplifying it. I urge you to make your self blank while understanding JavaScript classes(not only for this article).
We must understand that JavaScript is a functional programming language.
and to understand the paradigm of functional programming language I request you to go through this article. (I’ll wait)
Everything in JavaScript is a function.

ES6 introduced class keyword, prior to that constructor function and a factory function are used to implement class like behavior in JavaScript.
Please pay attention to the following example of each.

Constructor Function
A constructor function always starts with the upper case later by convention and we can create an instance of a constructor function using new keyword.
Due to these properties, we can achieve class like behavior in JavaScript using constructor function.


The Person function above provides almost similar functionality as of class in Java.
Every function has special property called prototype. this property is an object and often called as prototype object. By default this prototype object has a property called constructor which points back to our function.

We can add new properties to the Class Person using prototype.
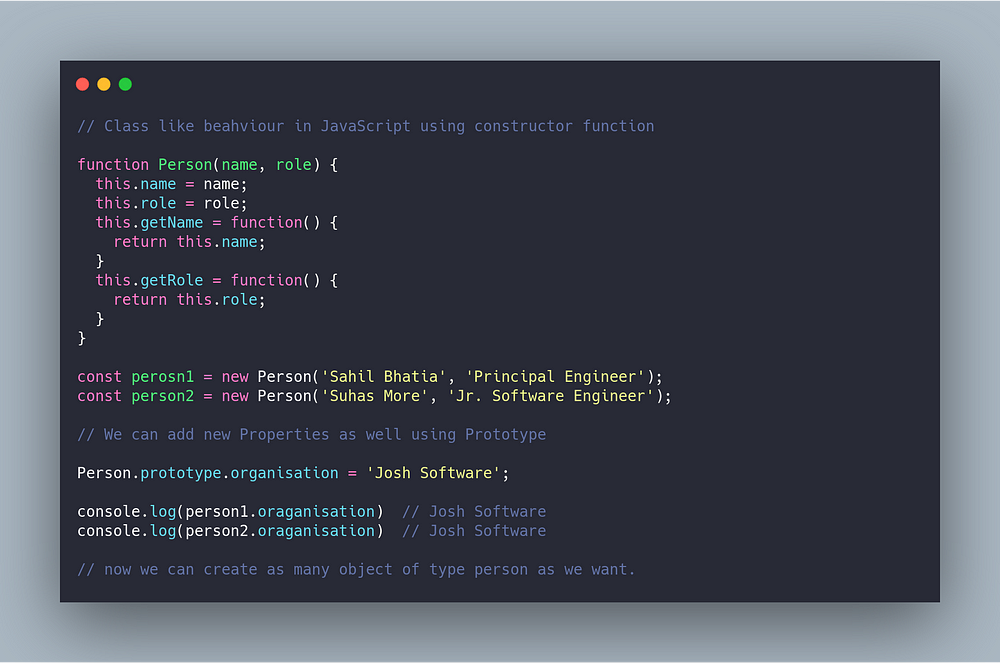
Properties added using prototype becomes automatically available to all the instances of the constructor function.

But it has one problem,
Reference type properties get shared between instances.

Now that we have understood constructor function and use of the prototype. Let’s understand what is Class keyword.
Class in JavaScript
You might think this is something different than constructor functions and prototypes, well it’s not!
Class is just syntactic sugar over constructor functions.
Don’t believe? I thought so, let’s have a look at the below example.
This is how we write a class in JavaScript.

And this is after transpiling the code to pure JavaScript, (heard Babel? good)

So the point is, Class keyword in JavaScript is just syntactic sugar for the sake of simplicity, under the hood it uses constructor function and power of prototype.
Well, this is just a tip of the iceberg, because real confusion is not over the implementation of a class, people get confused over this keyword, lexical scoping of this and so on.
For more deep understanding consider the following links.
this, this and this.
If you enjoyed this article, applaud and sharing is highly appreciated.
Follow me on Twitter @suhas0101